파일 찾기
특정 파일명을 입력받아 그 파일이 cpp 파일이 존재하는 현재 폴더에 있는지 확인하기
#include <windows.h>
#include <stdio.h>
#include <strsafe.h>
#include <tchar.h>
void findFirstFile(TCHAR *input) {
WIN32_FIND_DATA FindFileData;
HANDLE hFind;
_tprintf(TEXT("Target file is %s\n"), input);
hFind = FindFirstFileA(input, &FindFileData);
if (hFind == INVALID_HANDLE_VALUE)
{
_tprintf(TEXT("FindFirstFile failed (%d)\n"), GetLastError());
}
else
{
_tprintf(TEXT("The first file found is %s\n"), FindFileData.cFileName);
FindClose(hFind);
}
}
int main() {
TCHAR input[80];
scanf_s("%[^\n]", &input, sizeof(input));
findFirstFile(input);
}
파일이 있는지 확인하는 함수인 FindFirstFileA(=FindFirstFile)을 사용한다. 파라미터는 검색할 파일명과 파일의 정보를 담을 WIN32_FIND_DATA 구조체가 필요하다.
구조체의 세부 설명은
https://docs.microsoft.com/ko-kr/windows/win32/api/minwinbase/ns-minwinbase-win32_find_dataa
함수의 리턴값은 핸들로 받는데 오류가 있는지 확인하는 용도로 쓰인다.
*핸들 : 응용프로그램이 커널에 직접 접근하는것을 막고자 응용프로그램 대신에 커널에 접근한 응용프로그램 요청의 반환값
오류가 없다면 FindFileData의 파일 이름이 들어있는 변수인 cFileName을 출력한다.
cpp 폴더 안에 있는 a.txt를 입력했을때 출력결과
입력을 char가 아닌 TCHAR로 받아온건 문자에 유니코드가 포함될 수 있기 때문이고 _tprintf, TEXT 모두 마찬가지다.
scanf_s의 %s 대신 %[^\n]을 쓴것은 띄어쓰기도 입력받기 위함이다. 엔터를 제외하고 모두 입력받으란 뜻이다.
참고 : https://docs.microsoft.com/en-us/windows/win32/api/fileapi/nf-fileapi-findfirstfilea
폴더 내 파일목록 출력하기
cpp파일이 존재하는 현재 폴더의 파일 목록 출력하기
#include <windows.h>
#include <stdio.h>
#include <strsafe.h>
#include <tchar.h>
void findList(TCHAR *input) {
WIN32_FIND_DATA FindFileData;
HANDLE hFind;
_tprintf(TEXT("Target file is %s\n"), input);
StringCchCat(input, MAX_PATH, TEXT("*"));
hFind = FindFirstFileA(input, &FindFileData);
if (hFind == INVALID_HANDLE_VALUE)
{
_tprintf(TEXT("FindFirstFile failed (%d)\n"), GetLastError());
return;
}
do
{
if (FindFileData.dwFileAttributes & FILE_ATTRIBUTE_ARCHIVE) // 파일만 검색
{
_tprintf(TEXT("%s\n"), FindFileData.cFileName);
}
}
while (FindNextFile(hFind, &FindFileData) != 0);
FindClose(hFind);
}
int main() {
TCHAR input[80] = "";
//scanf_s("%[^\n]", &input, sizeof(input));
findList(input);
}
파일이 있는지 확인하는 함수인 FindFirstFileA를 실행 후 다음 파일을 검색하는 FindNextFile을 파일이 없을때까지 반복실행하여 모든 현재 폴더 내 모든 파일을 출력한다.
반복문에서 FindFileData.dwFileAttributes & FILE_ATTRIBUTE_ARCHIVE는 파일을 찾는 속성을 지정하는데
FILE_ATTRIBUTE_ARCHIVE 는 파일만 검색하는 것이고 (설명은 A file or directory that is an archive file or directory 라고 되어있는데 파일만 검색한다. 이유는 모르겠다.)
FILE_ATTRIBUTE_DIRECTORY 는 하위 폴더만 검색한다.
다른 속성은
https://docs.microsoft.com/ko-kr/windows/win32/fileio/file-attribute-constants
cpp 폴더에서 컴파일 했을때 결과
위에서는 입력받은 경로(input 초기화값)에 *를 붙여서 현재 폴더의 모든 파일을 확인한다.
MAX_PATH는 윈도우의 파일명 최대길이다.
만약
StringCchCat(input, MAX_PATH, TEXT("*")); 을
StringCchCat(input, MAX_PATH, TEXT("\\*")); 로 고치고 폴더명을 입력받으면
입력받은 폴더의 파일들을 출력할 수 있다.
그리고 특정 확장자만 출력하고 싶으면
StringCchCat(input, MAX_PATH, TEXT("\\*.txt")); 와 같이 수정하면 된다.
다른 글
2019/09/12 - [언어/C, C++] - [C++, Windows] 파일 이름 변경
[C++, Windows] 파일 이름 변경
파일 이름 변경 rename 함수사용 int rename( const char *oldname, const char *newname ); 반환값이 0이면 정상, -1이면 오류 #include void reName(char *input, char *newName) { /* Attempt to rename file:..
hydroponicglass.tistory.com
참고
https://docs.microsoft.com/ko-kr/windows/win32/fileio/listing-the-files-in-a-directory
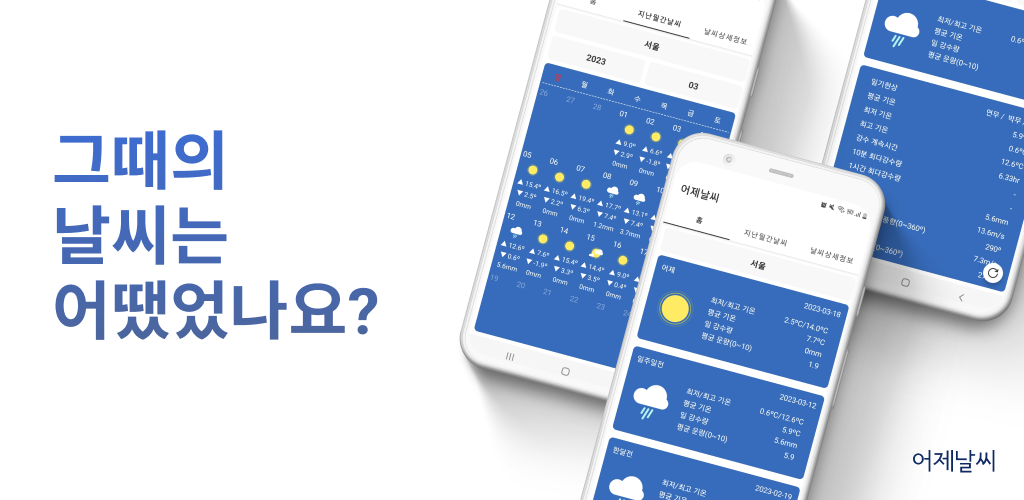
최근댓글