반응형
Bottom Sheet Dialog
Bottom Sheet Dialog는 하단에서 슬라이드 애니메이션으로 나타나는 다이얼로그다.
버튼을 선택시 BottomSheetDialog가 출력되고, BottomSheetDialog에서 ListView의 item을 선택하면 해당 Toast를 출력한다.
구현
themes.xml
Bottom Sheet Dialog 뒤 액티비티를 반투명 처리하는 테마를 사용하기 위해 아래 코드를 추가한다.
<style name="BottomSheetDialogTheme" parent="Theme.Design.BottomSheetDialog">
<item name="bottomSheetStyle">@style/BottomSheetStyle</item>
</style>
<style name="BottomSheetStyle" parent="Widget.Design.BottomSheet.Modal">
<item name="background">@android:color/transparent</item>
</style>
bottom_sheet_background.xml
Dialog의 색, 모양 등을 정한다.
Radius로 모서리를 라운딩처리한다.
<?xml version="1.0" encoding="utf-8"?>
<shape xmlns:android="http://schemas.android.com/apk/res/android"
android:shape="rectangle">
<solid android:color="@color/white"/>
<corners android:topLeftRadius="10dp"
android:topRightRadius="10dp"/>
</shape>
layout_bottom_sheet.xml
위에서 만든 bottom_sheet_background.xml을 배경으로 적용한다.
Dialog에 ListView를 추가한다.
Dialog에서 스크롤을 하기 위해 nestedScrollingEnabled를 true로 둔다.
<?xml version="1.0" encoding="utf-8"?>
<androidx.appcompat.widget.LinearLayoutCompat xmlns:android="http://schemas.android.com/apk/res/android"
...
android:background="@drawable/bottom_sheet_background">
<ListView
android:id="@+id/listView"
android:layout_width="match_parent"
android:layout_height="512dp"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintLeft_toLeftOf="parent"
android:nestedScrollingEnabled="true"/>
</androidx.appcompat.widget.LinearLayoutCompat>
layout_list_view_item.xml
리스트뷰에 출력되는 텍스트뷰를 추가한다.
<?xml version="1.0" encoding="utf-8"?>
<androidx.appcompat.widget.LinearLayoutCompat xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical">
<TextView
android:id="@+id/textView"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingVertical="16dp"
android:textColor="@color/black"
android:gravity="center"
android:text="text"/>
</androidx.appcompat.widget.LinearLayoutCompat>
activity_main.xml
Dialog를 출력하기 위한 버튼을 추가한다.
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
...
<androidx.appcompat.widget.AppCompatButton
android:id="@+id/button_select"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="button"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent"/>
</androidx.constraintlayout.widget.ConstraintLayout>
ListViewAdapter.kt
리스트뷰의 텍스트를 선택하면 토스트메세지를 출력한다.
해당 코드는 리스트뷰의 선행 이해가 필요하다. 전체코드는 아래 깃헙을 참고한다.
class ListViewAdapter (val context : Context,
val list : ArrayList<String>,
val bottomSheetDialog: BottomSheetDialog) : BaseAdapter()
{
...
override fun getView(p0: Int, p1: View?, p2: ViewGroup?): View {
val view : View = LayoutInflater.from(context).inflate(R.layout.layout_list_view_item, null)
val textView = view.findViewById<TextView>(R.id.textView)
val item = list[p0]
textView.setText(item)
// 리스트뷰의 textView 선택시 토스트 메세지 출력
textView.setOnClickListener{
Toast.makeText(context, item, Toast.LENGTH_SHORT).show()
}
return view
}
}
MainActivity.kt
버튼을 선택했을 때 다이얼로그를 출력한다.
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
...
// 버튼 선택
button_select.setOnClickListener {
// 리스트뷰에 출력할 리스트 생성
val list = ArrayList<String>()
list.add("1")
list.add("2")
list.add("3")
list.add("4")
// bottomSheetDialog 객체 생성
val bottomSheetDialog = BottomSheetDialog(
this, R.style.BottomSheetDialogTheme)
// layout_bottom_sheet를 뷰 객체로 생성
val bottomSheetView = LayoutInflater.from(applicationContext).inflate(
R.layout.layout_bottom_sheet, null)
// 리스트뷰어댑터 객체 생성
val listViewAdapter = ListViewAdapter(this, list, bottomSheetDialog)
// bottomSheetView의 리스트뷰에 생성한 리스트 연결
bottomSheetView.findViewById<ListView>(R.id.listView).adapter = listViewAdapter
// bottomSheetDialog 뷰 생성
bottomSheetDialog.setContentView(bottomSheetView)
// bottomSheetDialog 호출
bottomSheetDialog.show()
}
}
}
전체 코드
https://github.com/HydroponicGlass/2021_Example_Android/tree/main/BottomSheetDialog_ListView
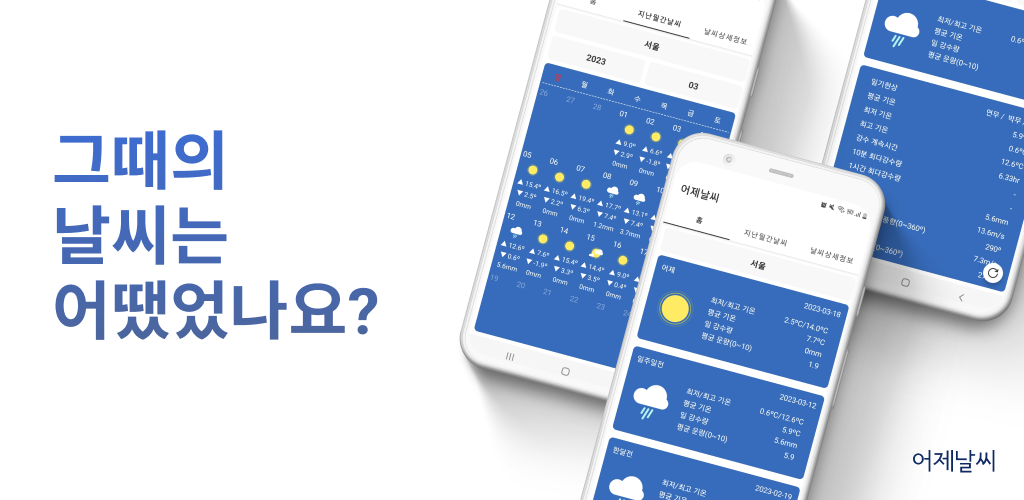
최근댓글