반응형
목적
버튼(Button) 클릭시 텍스트뷰(TextView)에 "Hello World"가 출력되게 한다.
본론
activity_main.xml
레이아웃에 버튼과 텍스트뷰를 그린다.
아래 activity_main.xml의 디자인과 코드는 예시이며 똑같이 만들 필요는 없다.
<?xml version="1.0" encoding="utf-8"?>
<!-- activity_main.xml -->
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<Button
android:id="@+id/button"
android:layout_width="173dp"
android:layout_height="87dp"
android:text="Button"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<TextView
android:id="@+id/textView"
android:layout_width="315dp"
android:layout_height="44dp"
android:gravity="center_horizontal"
android:lineSpacingExtra="18sp"
android:textAllCaps="false"
android:textAppearance="@style/TextAppearance.AppCompat.Display1"
android:textSize="24sp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/button" />
</androidx.constraintlayout.widget.ConstraintLayout>
MainActivity.kt
버튼 클릭시 이벤트 처리는 setOnClickListener를 이용한다.
이 글에서 이벤트는 텍스트뷰에 텍스트를 출력하는 것으로, 텍스트뷰 클래스의 setText 메서드를 이용한다.
setText는 텍스트를 텍스트뷰에 출력한다.
button.setOnClickListener{ // button : Button id
text.setText("Hello World") // text : TextView id
}
onCreate에 setOnClickListener를 구현한다.
xml에서 버튼의 id는 button, 텍스트뷰의 id는 textView로 지정했다.
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
val button : Button = findViewById(R.id.button)
val text : TextView = findViewById(R.id.textView)
button.setOnClickListener{
text.setText("Hello World")
}
}
버튼 클릭시 텍스트뷰에 "HelloWorld"가 출력되는 것을 확인할 수 있다.
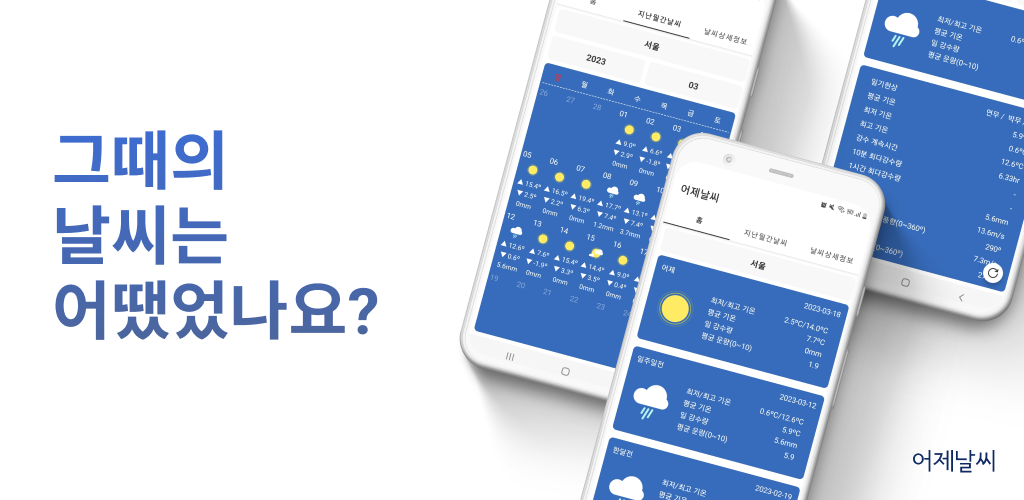
최근댓글